Creating a simple page in Drupal 7 & 8
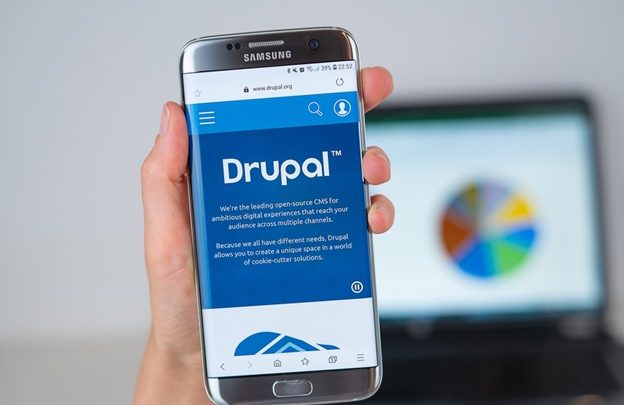
If you’re new to Drupal, it can be a bit daunting. It’s an inescapable CMS, running everything from banks to whole governments, but it’s also a big clunky machine with a lot of moving parts.
What you do get out of that is a flexibility and power you’re not going to see in a lot of other places. If you can grok Drupal, then you’ve got a powerful tool at your disposal. Where better to start than with a blank page?
Drupal 7
Set up your module (here we’re calling it test_page), then implement a hook menu using the following steps:
- Create a menu function inside the module file test_page.module
function test_page_menu() |
- Under that menu, create an array containing, at minimum: title, description, page callback (directed at the function output), and permissions.
{ $items = array (); $items [‘test’] = array ( ‘title’ => ‘A cool page’, ‘description’ => ‘I am making a blank page!’, ‘page callback’ => ‘test_page_output’, ‘access callback’ => TRUE, ); |
- Return and close the array
return $items; } |
- Create a page function that returns your page
}
function test_page_output() { |
Putting it all together:
function test_page_menu () { $items = array (); $items [‘test’] = array ( ‘title’ => ‘A cool page’, ‘description’ => ‘I am making a blank page!’, ‘page callback’ => ‘test_page_output’, ‘access callback’ => TRUE, ); return $items; }function test_page_output() { return ‘A cool page’; } |
Drupal 8
If you were fine with Drupal 7, you’re probably going to be fine with Drupal 8: YAML is a bit of a lifesaver, to be honest. You need the following:
- Create a file [name].routing.yml in the top-level module directory, like this:
test_page.routing.yml
- Create a path. This must open with a forward slash. You can do some fun stuff with dynamic properties in curly braces if you’re feeling brave, but we’re sticking to the basics for now:
path: ‘/test_page’
- Set your defaults. At minimum you need a page title and a controller. The structure for the controller path is \Drupal\{module name}\Controller\{Class Name}::{Method Name}
defaults: _title: ‘A cool page’ _controller: ‘\Drupal\test_page\Controller\TestPageController::page |
- Set your requirements, which govern who can access this particular page. There’s a couple of ways to do this, but we’re sticking with the simplest, which will let anybody view.
requirements:
_access: ‘TRUE’
Putting it all together, you get:
test_page.page:
path: ‘/test_page’
defaults:
_title: ‘A cool page’
_controller: ‘\Drupal\test_page\Controller\TestPageController::page
requirements:
_access: ‘TRUE’
And that does it for the yml file. Now, we need to make the controller file. You’ll need to use the “page” method in the above file.
<?php namespace Drupal\test_page\Controller; use Drupal\Core\Controller\ControllerBase;class TestPageController { public function page() { $pageElement = array ( ‘#markup’ => ‘This is a cool page’, ); return $pageElement; } } ?> |
You’ll also want an .info.yml file to tell drupal basic information about your module.
Make a file called test_page.info.yml in the root of your module folder, and place this inside of it.
name: Drupal Test Page description: A basic test page for Drupal 8 package: Customtype: module core: 8.x |
Finally, make a file called test_page.links.menu.yml and place the following inside of it
test_page.menu: title: ‘A Cool Page’ route_name: test_page.page |
And that’s it! You now know how to make simple, blank pages in Drupal 7 and 8. Now it’s time to start filling them! But that’s a guide for another day.
Want to read more? At this stage, Drupal 7 developers are probably going to want to brush up on PHP7 Error Reporting If you’re still thinking about your options, you might want to read about Drupal vs WordPress. If you’re thinking more about the business end of things, check out 5 Resume Tips For Software Developers.